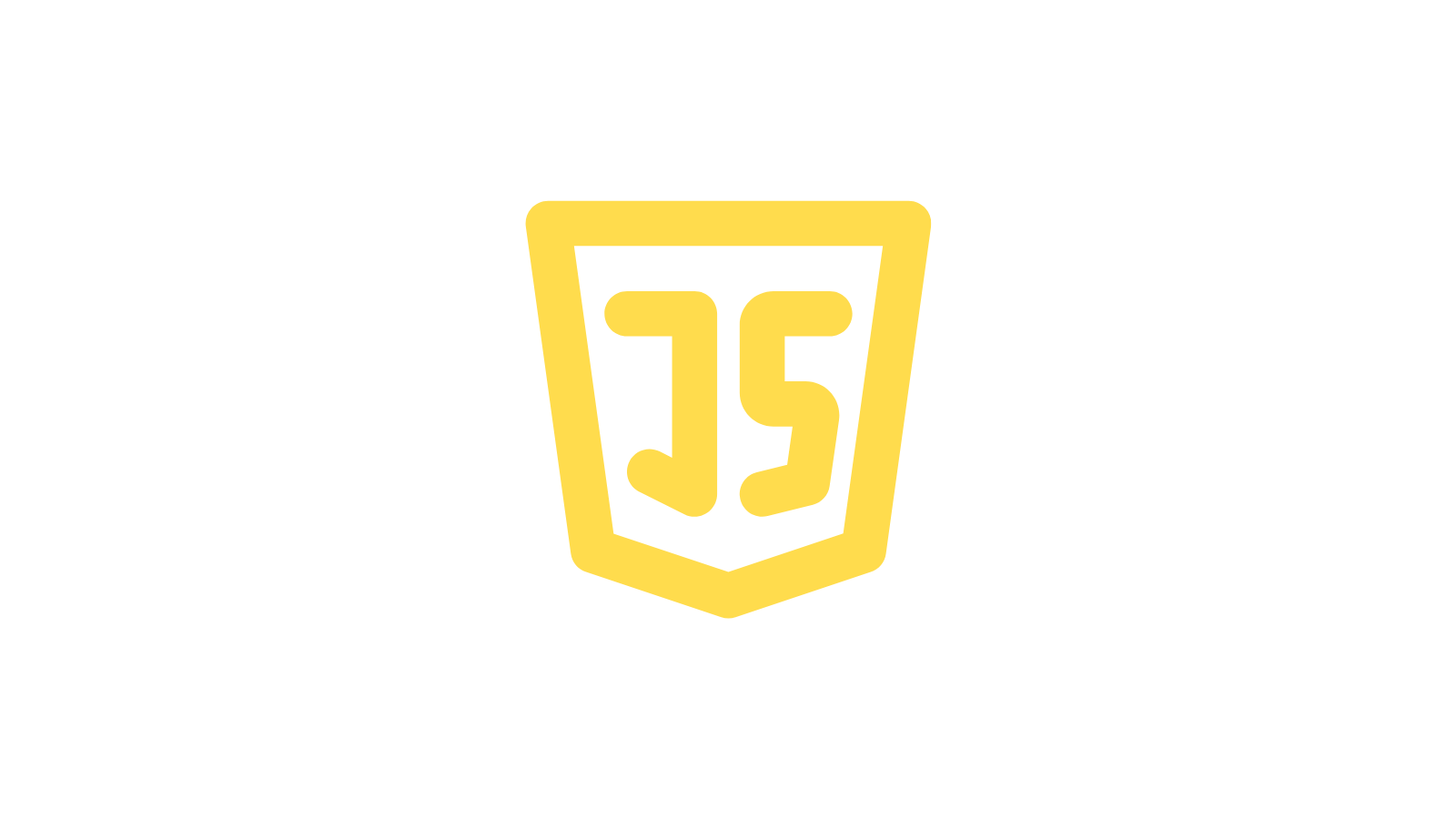
Learning JavaScript for Beginners
Difficulty: Easy
Posted: Jan 14th, 2025 | By the 404Found Team
JavaScript is a versatile programming language used for adding interactivity to web pages. It allows you to create dynamic and responsive user experiences.
What You’ll Learn:
- What JavaScript is and how to include it in your HTML.
- Basic JavaScript concepts like variables, functions, and events.
- How to write and execute your first JavaScript code.
Step 1: Adding JavaScript to Your HTML
You can include JavaScript in your HTML file using the <script>
tag. Here’s an example:
<script>
console.log("Hello, World!");
</script>
Step 2: Using External JavaScript Files
To keep your code organized, you can write JavaScript in an external file and link it to your HTML:
<script src="script.js"></script>
Save your JavaScript code in a file named script.js
and place it in the same directory as your HTML file.
Step 3: Writing Your First JavaScript Code
Here’s a simple example that displays an alert message when the page loads:
alert("Welcome to JavaScript!");
Step 4: Working with Variables
Variables store data that you can use later. Here's an example:
let name = "John";
console.log("Hello, " + name);
Step 5: Adding Interactivity with Events
JavaScript can respond to user actions, like clicking a button:
<button onclick="sayHello()">Click Me</button>
<script>
function sayHello() {
alert("Hello, World!");
}
</script>
Step 6: Exploring More Features
Now that you know the basics, try exploring:
- Loops and conditional statements for decision-making.
- Working with arrays and objects to manage data.
- Using the DOM (Document Object Model) to manipulate HTML elements.
Happy coding!
404Found Team
Posted: Jan 14th, 2025
At 404Found, we are committed to providing high-quality content that keeps you well-informed in the fast-paced and constantly evolving world of technology. Our goal is to ensure you stay up-to-date with the latest trends, innovations, and insights that shape the tech landscape.