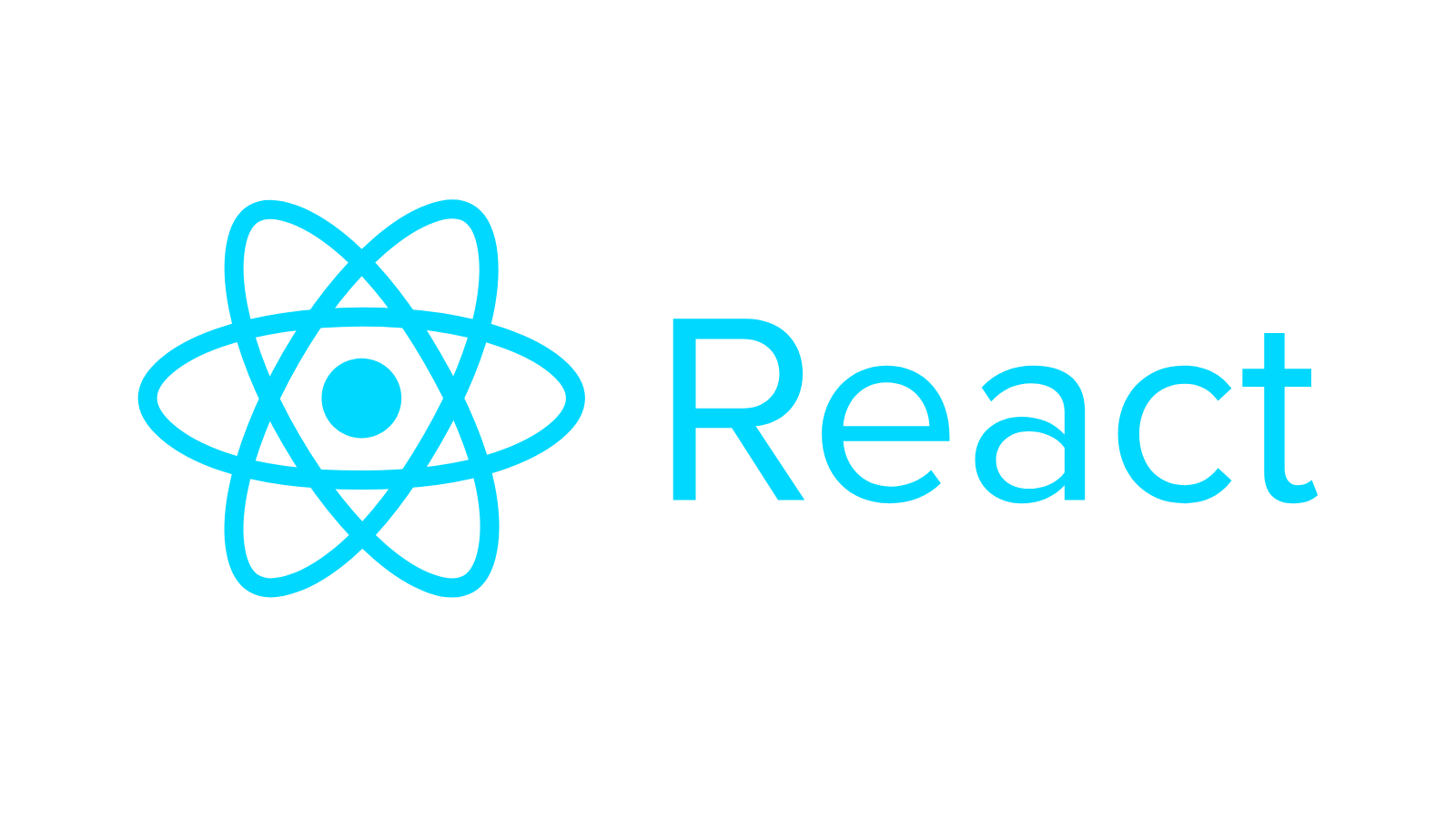
What is React?
React, or React.js, is a JavaScript library that helps developers build interactive and dynamic user interfaces (UI) for web applications. But let's break it down in a way that’s easy to understand—no tech jargon here, just pure fun!
The React Power-Up
Imagine you’re playing your favorite video game, and you’ve just unlocked a power-up that makes your character faster, more responsive, and cooler than ever. That’s exactly what React does for your web apps. It makes your user interface (UI) fast, efficient, and interactive. But how does it do that? Well, React uses a clever trick called a virtual DOM.
The Magic of Virtual DOM
Let’s say you're editing your Facebook profile, updating your status, or liking your friend's post. Normally, every tiny change would need the entire page to reload or re-render itself. With React’s virtual DOM, only the parts of the page that have changed will get updated, making the whole process lightning fast!
Think of it like this: Imagine trying to rearrange a bookshelf. If you had to take everything off the shelf, look at each book, and put them back one by one every time you move a book, that would be exhausting! But if you had a magic book-sorting robot that only removed and replaced the books that actually moved, you’d save a ton of time and energy. That's what React does, but for your app’s UI.
Component-Based Architecture
React doesn’t just make the UI faster—it organizes it! It’s built around something called components. Think of components as the building blocks of your app. Each component is like a mini app that controls a small piece of the interface, such as a button, a form, or a list of items.
For example, let's say you have a to-do list app. The whole page might have multiple components like the header, the task list, and the task item itself. The great part about React is that each component can be reused and customized however you like. Just like Lego blocks, you can snap together different pieces to build something truly awesome!
State and Props: The Dynamic Duo
Now, let's talk about two of React's most powerful features: state and props. These two work together like the best buddy team ever to make your app interactive and dynamic.
- State: Imagine you’re building a social media app where users can like posts. Every time a user clicks the "like" button, the number of likes should update. In React, state holds that information. It’s like a memory that remembers how many likes a post has.
- Props: Think of props as a way to pass information between components. It’s like giving your friend a note with instructions. For example, if your app’s parent component is managing a list of users, it might pass down the information about each user to a child component so it can display their name and profile picture.
React’s JSX: Writing HTML in JavaScript
Here’s a cool twist: React allows you to write HTML-like code directly inside your JavaScript code. This is called JSX (JavaScript XML). With JSX, you can describe the UI you want in a syntax that’s almost like HTML, but it still works seamlessly with JavaScript.
Here’s an example:
const button = ;
See what we did there? JSX makes it super easy to define the structure of your app and handle user interactions all in one place. It’s like combining the best of both worlds—HTML and JavaScript—into one powerful tool!
React Hooks: The Power of Simplicity
If you’ve been working with React for a while, you’ve probably heard of React Hooks. These nifty little functions were introduced to make it easier to manage state and lifecycle events in your components. Previously, you had to write class-based components, which could be complicated and long-winded. But with hooks, everything becomes simpler and more streamlined. It’s like switching from a manual transmission to an automatic car—less hassle, more fun!
Some popular hooks include:
- useState: This hook lets you add state to functional components.
- useEffect: This hook lets you perform side effects like fetching data, subscribing to a service, or updating the DOM.
- useContext: This hook lets you share data between components without passing props down manually.
Why Should You Learn React?
Now that you know the basics of what React is, you might be wondering, "Why should I learn it?" Well, let me give you a few good reasons:
- Fast Learning Curve: React is beginner-friendly! Its simple and declarative syntax makes it easier for you to learn and start building projects quickly.
- Huge Community and Ecosystem: React has one of the largest and most supportive communities of developers. That means if you ever run into a problem, chances are someone has already solved it for you!
- Reusable Components: React’s component-based structure lets you reuse code, which can save you a lot of time and effort. Plus, components are easy to maintain!
- Popular Frameworks and Tools: Because React is so widely used, many popular tools and frameworks have been built around it, including Next.js for server-side rendering and React Native for building mobile apps.
React in Action: Examples
Still not sure if React is right for you? Let’s take a look at some projects you can build using React!
- To-Do List App: Create an app where users can add, delete, and check off tasks. Use React to manage the state of the tasks and make the app interactive.
- Blog: Build a blog that fetches data from an API and displays posts dynamically. React will allow you to update the content without reloading the page.
- Weather App: Use React to display weather information from a public API. You can build a search bar and show current weather data based on the user’s location.
Conclusion: React—The Game-Changer for Web Development
React has completely changed the game when it comes to building dynamic and efficient web apps. From its fast rendering capabilities to its modular, component-based approach, React makes developing modern web apps easier and more fun. Whether you're a newbie or an experienced developer, React has something to offer. So, what are you waiting for? Start building with React today and watch your apps come to life!
And the best part? React doesn’t just help you build web apps—it helps you build apps that people love to use!
404Found Team
Posted: Jan 20th, 2025
At 404Found, we bring you the latest web development insights, tools, and trends. Our mission is to ensure you're always on top of the next big thing. Whether it's Web3, AI, or emerging tech, we've got you covered!